How to Script in Roblox for Absolute Beginners: Embark on a journey into the exciting world of Roblox scripting! This guide provides a friendly and comprehensive introduction to Lua programming within the Roblox Studio environment. We’ll cover everything from setting up your workspace and understanding fundamental concepts like variables and data types to mastering more advanced techniques such as event handling, object manipulation, and creating engaging game mechanics.
Whether you’re a complete novice or have some prior programming experience, this guide will equip you with the skills to bring your creative Roblox projects to life.
We will walk you through each step, offering clear explanations and practical examples. You’ll learn how to use LocalScripts and ServerScripts effectively, understand the importance of efficient code organization, and develop robust error-handling strategies. By the end of this guide, you’ll be confident in creating your own interactive experiences within the Roblox platform, ready to build games, tools, and more!
Introduction to Roblox Scripting: How To Script In Roblox For Absolute Beginners
Roblox scripting allows you to bring your creations to life by adding interactivity, dynamic elements, and complex game mechanics. This involves using the Lua programming language to control various aspects of your Roblox experiences. We’ll explore the fundamental concepts and techniques needed to start scripting in Roblox.
Roblox Script Types
Roblox offers two primary script types: LocalScripts and ServerScripts. Understanding their differences is crucial for building robust and secure games.
- LocalScripts: These scripts run on the client’s computer (the player’s machine). They are ideal for handling user interface elements, visual effects local to the player, and client-side logic. Changes made by a LocalScript are only visible to that specific player.
- ServerScripts: These scripts run on Roblox’s servers. They are responsible for managing game logic, handling data persistence, and ensuring fair gameplay across all players. ServerScripts control the game’s core mechanics and data, preventing cheating and ensuring consistency.
Overview of Lua
Lua is a lightweight, high-level programming language known for its simplicity and ease of use. It’s the language used for Roblox scripting. Lua features include variables, functions, loops, and conditional statements, providing the tools to create complex game logic.
“Hello, World!” Script
Let’s start with a classic: the “Hello, World!” program. This simple script demonstrates the basic structure of a Roblox script.
Line Number | Code | Explanation |
---|---|---|
1 | print("Hello, World!") |
This line uses the print() function to display the text “Hello, World!” in the Roblox Studio output window. |
Setting up Your Roblox Studio Environment
Before you start scripting, you need to familiarize yourself with the Roblox Studio interface. This section guides you through setting up your workspace and creating your first script.
Roblox Studio Interface
Roblox Studio’s interface comprises several key components: the Explorer window (for managing game objects), the Properties window (for modifying object properties), and the Script Editor (for writing and editing scripts). The 3D viewport displays your game world in real-time, allowing you to visualize your creations.
Creating a New Place and Inserting a Part
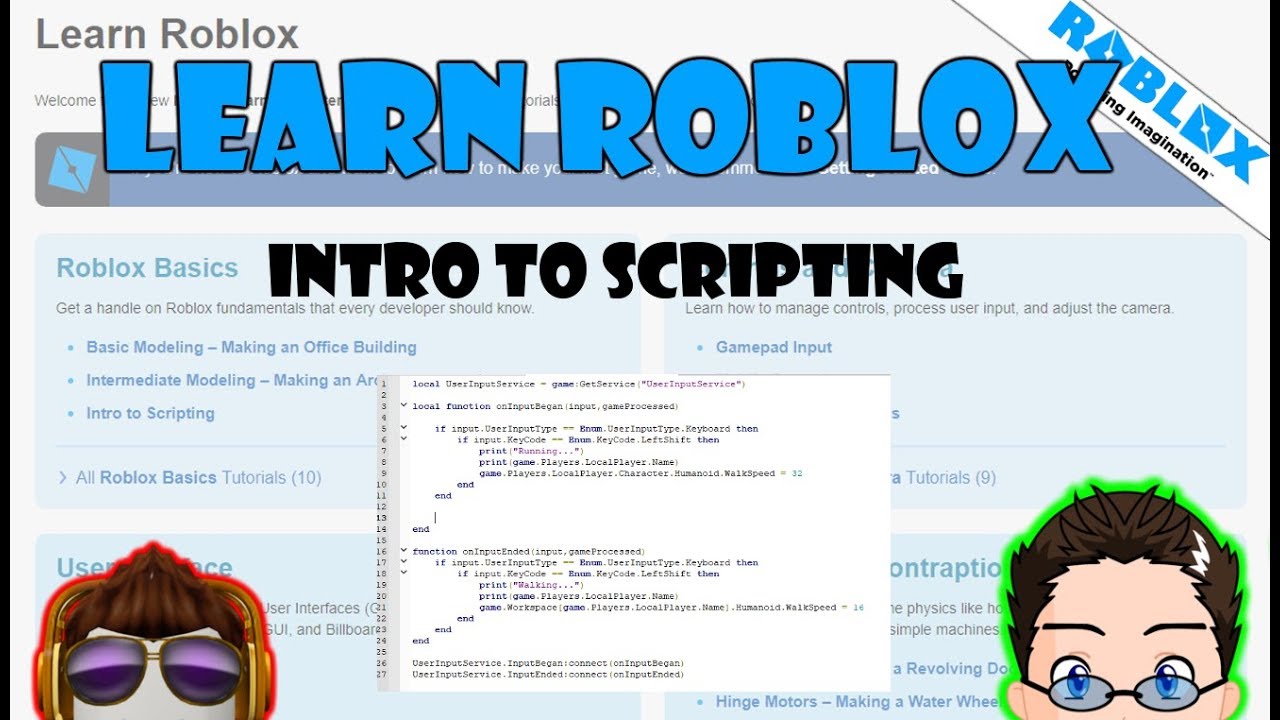
Source: ytimg.com
To begin, create a new Roblox place. Then, insert a simple part (a cube) into the workspace. This provides a basic object to interact with using your scripts. You can do this by navigating to the “Insert” menu and selecting “Part”.
Opening the Script Editor
Right-click on the part you just inserted in the Explorer window. Select “Insert Object” and then choose “Script”. This opens the Script Editor, where you can write your Lua code. Alternatively, you can drag and drop a Script object from the toolbox onto the part.
Organizing Scripts
For larger projects, organizing your scripts is crucial. Use descriptive names for your scripts and consider grouping related scripts into folders within the Explorer window. This improves readability and maintainability.
Understanding Variables and Data Types
Variables are fundamental to programming. They act as containers for storing data, allowing you to manipulate and reuse information within your scripts. Lua supports several data types.
Variables in Lua
Variables in Lua are declared using the assignment operator (=). No explicit type declaration is needed; Lua infers the type based on the assigned value.
Data Types
Lua’s primary data types include:
- Number: Represents numerical values (e.g., 10, 3.14).
- String: Represents text enclosed in double quotes (e.g., “Hello”).
- Boolean: Represents true or false values.
Variable Examples
Here are examples of declaring and using variables:
Code | Explanation |
---|---|
local myNumber = 10 local myString = "Hello, Roblox!" local myBoolean = true |
Declares a number, string, and boolean variable. The local indicates that the variable is only accessible within the current scope. |
Script with Data Types and Calculations
This script demonstrates basic calculations using different data types:
local num1 = 5 local num2 = 10 local sum = num1 + num2 print("The sum is: " .. sum) |
Performs addition and prints the result. The “..” operator concatenates strings. |
Working with Events and Functions
Events and functions are essential for creating interactive experiences. Events trigger actions based on specific occurrences, while functions encapsulate reusable blocks of code.
Events in Roblox Scripting
Roblox uses events to signal when something happens in the game, such as a player clicking a button or an object being touched. Scripts can listen for these events and execute code in response.
Event Listeners
Event listeners are used to respond to events. For instance, Touched
event fires when an object is touched, and MouseButton1Click
fires when the left mouse button is clicked on a part.
Creating and Calling Functions
Functions group related code together, making scripts more organized and reusable. They are defined using the function
.
function myFunction() print("Function called!") end myFunction() |
Defines a function and calls it. |
Script Responding to User Click
This script changes a part’s color when clicked:
local part = script.Parent part.MouseButton1Click:Connect(function() part.BrickColor = BrickColor.new("Bright red") end) |
Connects a function to the MouseButton1Click event of the part. |
Manipulating Objects and Properties
Roblox objects (like Parts, Bricks, and GUI elements) have properties that define their appearance and behavior. You can access and modify these properties using Lua scripts.
Accessing and Modifying Properties
Properties are accessed using the dot operator (.). For example, part.Position
accesses the part’s position.
Using Instance.new()
The Instance.new()
function creates new objects in the game. This is how you dynamically add objects during runtime.
Changing Properties Based on User Input
You can create interactive elements by changing object properties in response to user actions, such as button clicks.
Script Changing Part Color on Button Click
This script uses a button to change a part’s color:
local part = workspace.Part local button = script.Parent button.MouseButton1Click:Connect(function() part.BrickColor = BrickColor.new("Bright blue") end) |
The button click changes the part’s BrickColor. |
Introduction to Loops and Conditional Statements
Loops and conditional statements are crucial for controlling the flow of your scripts, allowing you to repeat actions and make decisions based on conditions.
For and While Loops
for
loops iterate a specific number of times, while while
loops continue as long as a condition is true.
If, Elseif, and Else Statements
These statements allow you to execute different blocks of code based on whether a condition is true or false.
Examples of Loops
A for
loop example:
for i = 1, 10 do print(i) end |
Prints numbers 1 through 10. |
Script Using Loops and Conditional Statements
This script demonstrates a simple scenario:
local part = script.Parent local count = 0 while count < 5 do part.Position = part.Position + Vector3.new(0, 1, 0) count = count + 1 wait(1) end |
Moves the part upwards 5 times with a 1-second delay. |
Working with Modules and Libraries
Modules and libraries promote code reusability and organization, especially in larger projects. They allow you to break down complex tasks into smaller, manageable units.
Modules and Their Benefits
Modules are self-contained units of code that can be easily reused across multiple scripts. They improve code organization and reduce redundancy.
Creating and Using Custom Modules
To create a module, save your code as a `.lua` file in Roblox Studio's ServerScriptService. Then, require it in other scripts using require()
.
Built-in Roblox Libraries
Roblox provides several built-in libraries that offer pre-built functions for common tasks, such as mathematical operations and string manipulation.
Modular Design Advantages
Modular design makes your code easier to maintain, debug, and extend. It also allows multiple developers to work on different parts of a project simultaneously.
Debugging and Troubleshooting
Debugging is an essential skill for any programmer. This section covers common errors and techniques for finding and fixing them.
Common Scripting Errors
Common errors include syntax errors (incorrect code structure), runtime errors (errors occurring during execution), and logical errors (incorrect program behavior).
Using Print Statements, How to script in roblox for absolute beginners
print()
statements are invaluable for debugging. They allow you to display variable values and track the flow of your script's execution.
Roblox Studio's Debugging Tools
Roblox Studio provides a debugger that allows you to step through your code line by line, inspect variables, and set breakpoints.
Troubleshooting Strategies
Effective troubleshooting involves systematically identifying the source of the error, testing changes incrementally, and using logging to track down issues.
Creating a Simple Game Mechanic
Let's design a simple points system for a game. This illustrates how to combine the concepts learned so far to create a functional game mechanic.
Points System Script
This script tracks points and updates a GUI element:
local points = 0 local pointsLabel = game.Players.LocalPlayer.PlayerGui.PointsLabel game.Workspace.PointSource.Touched:Connect(function() points = points + 1 pointsLabel.Text = "Points: " .. points end) |
Touching the PointSource adds a point and updates the label. |
Game Mechanic Flow
- Player touches the PointSource.
- The
Touched
event fires. - The points variable increments.
- The pointsLabel GUI is updated to display the new points total.
Advanced Concepts (Optional)
This section briefly introduces more advanced topics for those wanting to delve deeper into Roblox scripting.
Object-Oriented Programming (OOP)
OOP is a powerful programming paradigm that involves organizing code around objects that contain data and methods. It's useful for creating complex and maintainable systems.
OOP Example
A basic example of OOP would involve creating a "Player" object with properties like health and methods like "takeDamage".
Coroutines for Asynchronous Operations
Coroutines allow you to run multiple tasks concurrently, which is beneficial for handling long-running operations without blocking the main thread.
Further Learning Resources
Roblox's developer hub, online tutorials, and community forums offer extensive resources for advanced learning.
Wrap-Up
Creating engaging and interactive experiences within Roblox is now within your reach! This guide has provided you with the foundational knowledge and practical skills necessary to embark on your Roblox scripting journey. From the simple "Hello, World!" script to more complex game mechanics, you've learned the core principles of Lua programming within the Roblox Studio environment. Remember that practice is key; the more you experiment and build, the more confident and proficient you will become.
So, dive in, explore the possibilities, and let your creativity shine within the vast and exciting world of Roblox development. Happy scripting!
Common Queries
What version of Roblox Studio is recommended for beginners?
The latest stable version of Roblox Studio is generally recommended. It offers the most up-to-date features and bug fixes.
Where can I find additional resources for learning Roblox scripting?
The Roblox Developer Hub is an excellent resource, offering comprehensive documentation, tutorials, and community forums. Numerous online courses and YouTube channels also provide valuable learning materials.
How do I save my Roblox scripts?
Save your scripts regularly by clicking "Save" in the Roblox Studio menu or using keyboard shortcuts (Ctrl+S or Cmd+S).
What are some common mistakes beginners make when scripting in Roblox?
Common mistakes include typos in variable names, incorrect syntax, and forgetting to handle potential errors. Careful attention to detail and consistent testing are crucial.